My Research
Arbitrary Mapping Algorithm
Introduction:
This project is a simple algorithm to re-arrange the values randomly in an array. I have come across many situations where I needed to implement different scenarios in each iteration or each time I run the application. And I have used several algorithms that are already available. But why not try something by myself? The sole purpose of sharing this is to not imply this algorithm is faster than others but to give you more options to choose from.
Background idea:
Not everything we do is logical. Let's say your friend asked you to buy him a bottle of water. You went to a shop and you found multiple same brand water bottles in a single row. What will you do in this scenario? As a member of the same species as you, my best guess is you will pick one randomly. Or let's think about the lottery draw. How do they select the winner? Yes, it's up to luck but in a logical language, we call it random. Computers are made to make our work easier and so it has to be programmed like the way we do the work.
Application:
This can have multiple applications based on the software it is being used for. I'll just bring some examples here to make the concept clear between us.
Let every index of the array color represents a unique color code.
String[] color = {"#67e11f", "#7429a3", "#4171cd", "#f3fad0"}; //HEX colour codes are stored as a string
and these colour represents the colour of 4 boxes. And your goal is to make an application which gives you different color on each start like the image 1.1.
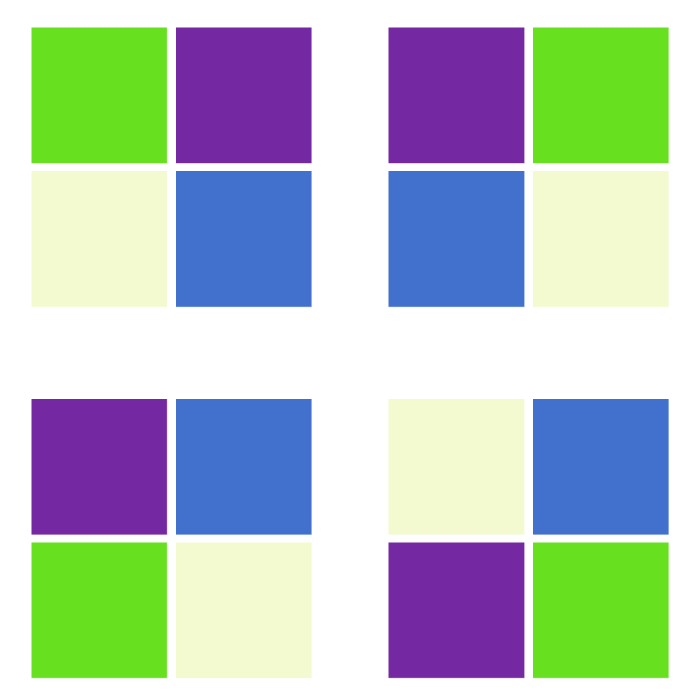
As you can see, each whole square (a combination of 4 small squares) represents each time you entered your application. And it is showing you different colors. So instead of having the same constant colour each time it is giving variation. Awesome! isn't it? So your possible color in the color[i]
will be any from color[0]
to color[SIZE-1]
. SIZE
is the length of the array.
Pseudocode (Java):
This pseudocode is asuming the data-type is String. But it will work with any data-type you want.
public String[] makeRandom(String[] color){
final int SIZE = color.length(); //size of color
final int RANDOM_CONSTANT = (int)*Math.random()*SIZE; //see Analysis section for details
String temporaryArray[] = new String[SIZE];
//see details in Analysis
for(i is from 0 to SIZE-1){
int index = (i+RANDOM_CONSTANT)%SIZE; //new random index
temporaryArray[i] = color[index];
}
return temporaryArray;
}
Analysis:
We can divide the algorithm into two major parts.
1. creating the RANDOM_CONSTANT
value.
2. Creating random index & passing the value
Math.random()
always returns 0.0 <= value < 1.0
. But we need to find a way make it an integer number between 0
upto the SIZE
. Solution is first multiply the number returned by random with the SIZE
. Now we have a number within our expected range (0 - SIZE
). Cast the number to an int. So we get:
final int RANDOM_CONSTANT = (int)*Math.random()*SIZE;
Create a loop same size as the color array. In each iteration the loop will create a random index value and assign it to a temporary array. For the new random index first we add the RANDOM_CONSTANT
with current index i
.But the problem happens now is it goes outside the scope of color array. The solution is modulo(%
). It helps the value keep in range (0<=i<SIZE
). So we get:
int index = (i+RANDOM_CONSTANT)%SIZE; //the new random index
Now that we have the random index, we will pass the value of color[index]
into the temporaryArray[i]
.temporaryArray[i]=color[index]; //passing the value
Now create a new loop and copy the temporaryArray values into color array. The values are noe randomized.
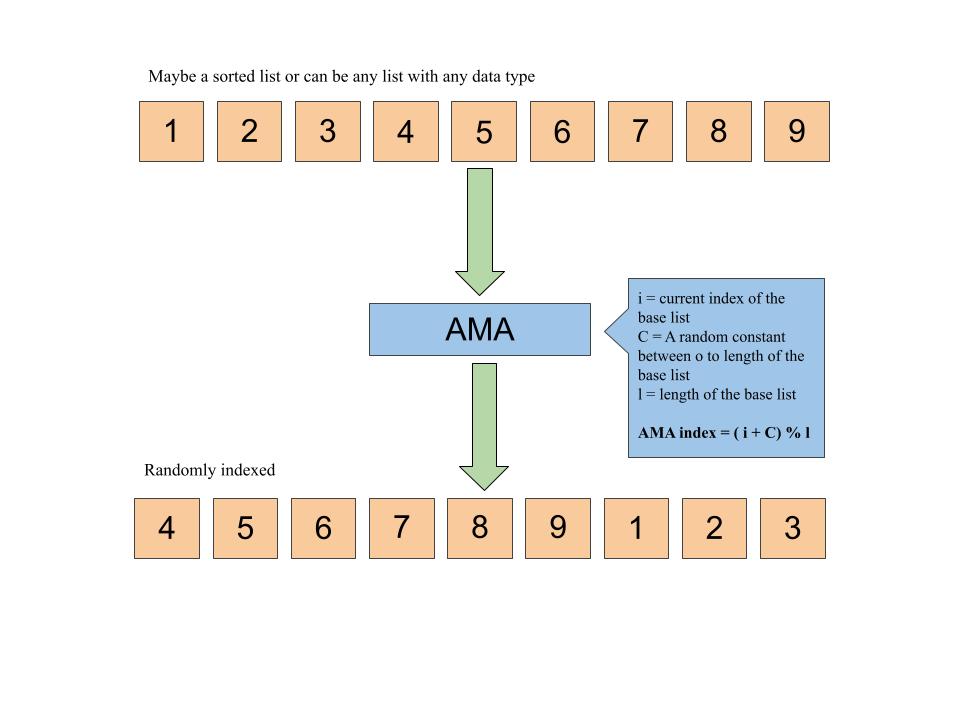
Limitation:
This algorithm has been tested only using the Java programming language. This does not indicate it won't work with other languages but also doesn't guarantee.
code: source
Author & Date: Jayed Rafi / August 2021